Notice
Recent Posts
Recent Comments
Link
250x250
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | 6 | 7 |
8 | 9 | 10 | 11 | 12 | 13 | 14 |
15 | 16 | 17 | 18 | 19 | 20 | 21 |
22 | 23 | 24 | 25 | 26 | 27 | 28 |
29 | 30 |
Tags
- 리눅스
- 공부
- 백준
- 학습
- 운영체제
- 백준알고리즘
- c
- 알고리즘
- 회귀
- Windows 10
- OpenCV
- Windows10
- 딥러닝
- CV
- 턱걸이
- 쉘
- 텐서플로우
- linux
- TensorFlow
- C언어
- python
- 시스템프로그래밍
- 코딩
- 영상처리
- shell
- Computer Vision
- error
- C++
- 프로그래밍
- 프로세스
Archives
- Today
- Total
줘이리의 인생적기
C - 연산자 본문
728x90
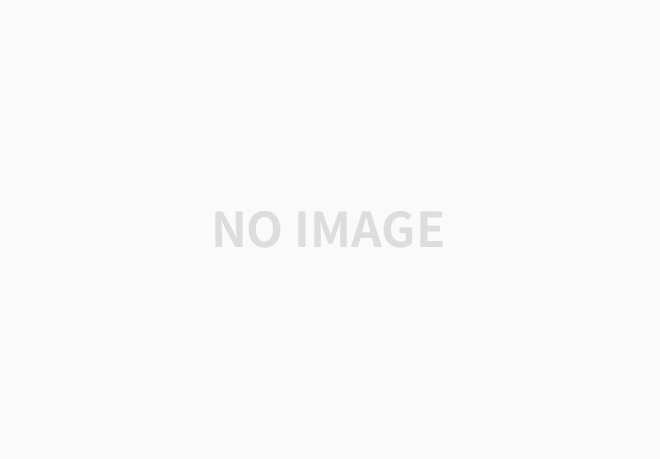
Index
- 산술 연산자, 대입 연산자
- 증감 연산자
- 관계 연산자
- 논리 연산자
- 형 변환 연산자
- sizeof
- 조건 연산자
1. 산술 연산자, 대입 연산자
예제로 설명 생략.
산술 연산자, 대입 연산자 예제
#include <stdio.h>
int main(void){
//산술 연산자
int a, b;
int sum, sub, mul;
a = 5;
b = 10;
sum = a + b;
sub = a - b;
mul = a * b;
printf("합은 %d, 차는 %d, 곱은 %d\n", sum, sub, mul);
//나누기, 나머지 연산자
int div, res;
div = b/3;
res = b%3;
printf("10/3의 몫은 %d, 나머지는 %d", div, res);
return 0;
}
실행결과(충분한 생각을 가진 후 누르기)
2. 증감 연산자
전위형, 후위형 증감연산자 포함 예제로 설명 생략.
증감 연산자 예제
#include <stdio.h>
int main(void){
//첫번째 예제
int a = 10, b = 10;
a++;
b--;
printf("a : %d, b : %d\n", a, b);
//두번째 예제
int c = 5, d = 5;
int pre, post;
pre = (++c)*5;
post = (d++)*5;
printf("c = %d, d = %d\n", c, d);
printf("전위형 (++c)*5 = %d, 후위형 (d++)*5 = %d", pre, post);
return 0;
}
실행결과(충분한 생각을 가진 후 누르기)
3. 관계 연산자
대소 관계 연산자(<, >)와 동등 관계 연산자(==, !=)가 존재
관계 연산자 예제
#include <stdio.h>
int main() {
int number = 5;
printf("number 가 4보다 크다 %d\n", number > 4);
printf("number 가 5보다 크다 %d\n", number > 5);
printf("number 가 10보다 크다 %d\n", number > 10);
printf("number 가 10보다 작다 %d\n", number < 10);
printf("number 가 5보다 작다 %d\n", number < 5);
printf("number 가 4보다 작다 %d\n", number < 4);
printf("number 가 5보다 크거나 같다 %d\n", number >= 5);
printf("number 가 5보다 작거나 같다 %d\n", number <= 5);
printf("number 는 5와 같다 %d\n", number == 5);
printf("number 는 4와 같다 %d\n", number == 4);
printf("number 는 5와 같지 않다 %d\n", number != 5);
printf("number 는 4와 같지 않다 %d\n", number != 4);
return 0;
}
실행결과(충분한 생각을 가진 후 누르기)
4. 논리 연산자
&&(and), ||(or), !(not)
논리 연산자 예제
#include <stdio.h>
int main() {
int a = 30;
int res;
res = (a > 10) && (a < 20);
printf("(a > 10) && (a < 20) : %d\n", res);
res = (a < 10) || (a > 20);
printf("(a > 10) && (a < 20) : %d\n", res);
res = !(a >= 20);
printf("! (a >= 20) : %d\n", res);
return 0;
}
실행결과(충분한 생각을 가진 후 누르기)
5. 형 변환 연산자
컴파일러의 형변환 기본규칙은 큰 값을 변환한다.
뭔소리냐면
형 변환 예제
#include <stdio.h>
int main() {
int a = 20, b= 3;
double res;
res = (a/b);
printf ("%d\n", res);
printf ("%lf\n", res);
//강제 형 변환
res = ((double)a) / ((double)b);
printf ("%lf\n", res);
return 0;
}
실행결과(충분한 생각을 가진 후 누르기)
6. sizeof
피연산자의 크기를 바이트 단위로 계산해서 알려준다
sizeof 예제
#include <stdio.h>
int main() {
printf("%d\n", sizeof(int));
printf("%d\n", sizeof(double));
printf("%d\n", sizeof(char));
return 0;
}
실행결과(충분한 생각을 가진 후 누르기)
7. 조건 연산자
조건 연산자 = 삼항 연산자
사용 방법만 알아보고 예제로 알아보자
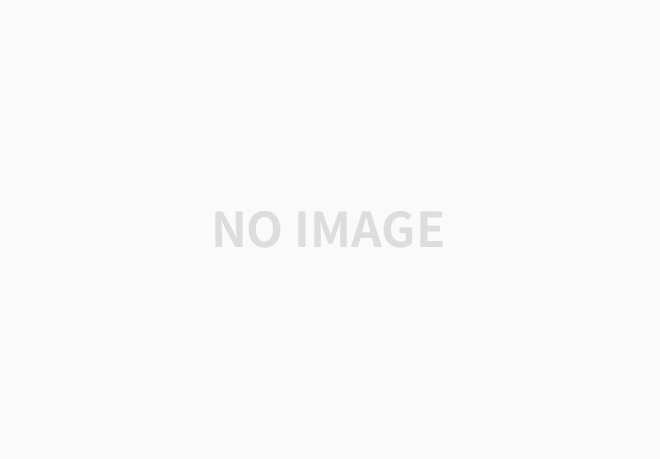
조건 연산자 예제
#include <stdio.h>
int main() {
int a = 10, b= 20;
int res;
res = (a > b) ? a : b;
printf("a 와 b 중 큰 값 = %d", res);
return 0;
}
실행결과(충분한 생각을 가진 후 누르기)
지적 및 개선사항은 언제든지 댓글로 부탁드립니다!
'공부 > C' 카테고리의 다른 글
C - 함수 (0) | 2020.05.12 |
---|---|
C - 반복문(while, for, do ~ while) (0) | 2020.05.12 |
C - 조건문(if, switch ~ case) (0) | 2020.05.12 |
C - 변수와 데이터 입력 (0) | 2020.05.12 |
C - 상수와 데이터 출력 (0) | 2020.05.11 |